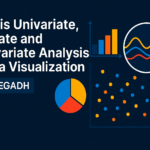
Student Result Management System in Python With Code
In today’s educational landscape, technology plays a vital role in streamlining processes that once required significant time and effort. One such area is student result management, where schools and colleges are shifting from manual to digital systems to store, process, and display student results efficiently. A Student Result Management System in Python is a great example of how programming can ease this critical process and empower institutions with a streamlined, error-free way to handle students’ academic data.
Building such a system in Python gives aspiring developers a unique opportunity to learn programming while creating something meaningful. In this blog, we’ll explore what a Student Result Management System is, why it matters, and how you can build one yourself with Python, complete with source code.
These problems are resolved by a student Result Management System through:
- Automating the result processing.
- Reducing errors in data entry and result calculation.
- Allowing easy access to students’ academic performance.
- Saving educators and Administrators time and energy.
Features
To make the system truly functional, it needs to include key features like:
- Student Data Management: Add, modify, and delete student records.
- Marks Entry: Input the marks for different subjects.
- Result Calculation: Automatically calculate results and grades.
- Result Display: Present results in a student-friendly format.
- Search and Filter: Look up students using their name, roll number, or other details.
- Data Security: Ensure only authorized personnel can access or modify student records.
Blood Pressure Monitoring Management System Using PHP and MySQL with Guide
Building a Simple Student Result Management System in Python
Step 1: Define the Student Class
We’ll start by defining a Student
class that holds individual student details like their name, roll number, and marks. This class will also include methods to calculate and display results.
class Student:
def __init__(self, roll_number, name, marks):
self.roll_number = roll_number
self.name = name
self.marks = marks # Dictionary to store subject-wise marks
def calculate_total(self):
"""Calculate the total marks of the student."""
return sum(self.marks.values())
def calculate_percentage(self):
"""Calculate the percentage based on the total marks."""
total_marks = self.calculate_total()
return (total_marks / (len(self.marks) * 100)) * 100
def display_result(self):
"""Display the student's result with total marks and percentage."""
print(f"Roll Number: {self.roll_number}")
print(f"Name: {self.name}")
print("Marks:")
for subject, mark in self.marks.items():
print(f"{subject}: {mark}")
print(f"Total Marks: {self.calculate_total()}")
print(f"Percentage: {self.calculate_percentage()}%\n")
This stage involves creating a Student class and initializing it with the roll number, name, and grades of a student. The class also includes methods to calculate total marks, percentage, and display the result.
Step 2: Adding the Student Management System
Next, we’ll create a system to add multiple students and manage their results. This is where we’ll handle operations like adding student data and viewing results.
class ResultManagementSystem:
def __init__(self):
self.students = [] # List to store student records
def add_student(self, roll_number, name, marks):
"""Add a new student to the system."""
student = Student(roll_number, name, marks)
self.students.append(student)
def display_all_results(self):
"""Display results of all students."""
if len(self.students) == 0:
print("No students in the system.")
else:
for student in self.students:
student.display_result()
def find_student(self, roll_number):
"""Find and display a student's result by roll number."""
for student in self.students:
if student.roll_number == roll_number:
student.display_result()
return
print(f"Student with roll number {roll_number} not found.")
The ResultManagementSystem
class acts as a manager for multiple students. It allows us to add new students, display all results, and find specific students by roll number.
Step 3: The User Interface (Main Program)
Finally, we’ll create a simple user interface where administrators can add students, view all results, or search for a specific student by roll number.
def main():
system = ResultManagementSystem()
while True:
print("\n--- Student Result Management System ---")
print("1. Add Student")
print("2. Display All Results")
print("3. Find Student by Roll Number")
print("4. Exit")
choice = input("Enter your choice: ")
if choice == '1':
roll_number = input("Enter Roll Number: ")
name = input("Enter Name: ")
subjects = int(input("Enter number of subjects: "))
marks = {}
for _ in range(subjects):
subject = input("Enter Subject Name: ")
mark = int(input(f"Enter Marks for {subject}: "))
marks[subject] = mark
system.add_student(roll_number, name, marks)
print("Student added successfully!")
elif choice == '2':
system.display_all_results()
elif choice == '3':
roll_number = input("Enter Roll Number to find: ")
system.find_student(roll_number)
elif choice == '4':
print("Exiting the system.")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
On this user interface, you are able to:
- Add a student: Enter details like roll number, name, and marks for various subjects.
- View all results: Display the results of all students in the system.
- Find a specific student: Search for a student’s results by roll number.
- Exit: Exit the program when done.
How the System Works
The Student Result Management System we’ve built in Python allows you to:
- Add new student records: You can input the student’s roll number, name, and marks for multiple subjects.
- View all students’ results: A simple command will display the total marks and percentage for all students stored in the system.
- Search for a specific student: Quickly find a student’s record by entering their roll number.
- Exit gracefully: The system exits when the user chooses the option.
Why This Project is Great for Learning
This project not only gives you hands-on experience in Python programming but also helps you understand:
- Object-Oriented Programming (OOP): We use classes to represent students and the system, encapsulating functionality and data.
- User Input and Data Processing: The project allows users to input multiple records, and the system handles all the processing.
- Error Handling: While simple, this system can be expanded to handle more complex error cases like invalid marks, duplicate students, etc.
- Scalability: This system can be extended with features like a database, graphical user interface (GUI), or even web-based access for real-time result checking.
student management system project in python code,
student management system project report in python pdf,
student management system project in python with mysql,
student result management system project report pdf,
student management system project in python with source code pdf,
student management system project in python class 12 pdf,
student-management system project in python with source code github,
student management system project in python ppt,
student result management system in python with source code,
student result management system in python pdf,
student result management system in python example,
student result management system in python github,
New Project :-https://www.youtube.com/@Decodeit2
1 comment