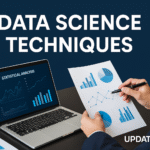
Currency Converter in Java Using Swing ,Step by Step-3 steps Free Source code
Currency Converter in Java
Introduction:
Introduction: In this tutorial, we will show you how to create a Currency Converter using the Java Swing library. You can convert currencies using this Currency Converter by using a graphical user interface (GUI) program. This program is built with the Java Swing package, which provides a comprehensive set of components for creating a graphical user interface (GUI). This application has a straightforward design with a button that converts the entered currency amount into the desired currency. So take a seat, get yourself a cup of coffee, and begin using the currency converter.
In this “Currency Converter in Java“ project, we use some Swing Components are Listed below
1) JPanel: JPanel is the Swing equivalent of the AWT class Panel, with an equivalent default layout.
2) The FlowLayout. JPanel is a direct descendant of JComponent.
3) JFrame: JFrame is Swing’s version of Frame and is a direct descendant of the AWT Frame class. The component added to the Frame is referred to as its Content.
4) JLabel: JLabel is a descendant of JComponent and is used to create text labels.
5) JButton: The JButton class handles push-button functionality. JButton lets you to associate a button with an icon, a string, or both.
6) JTextField: JTextFields allow you to alter a single line of text.
here’s a step-by-step explanation of the code, including each line of code:
Import necessary libraries
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.DecimalFormat;
- We import the required Java Swing libraries for creating the graphical user interface.
DecimalFormat
is imported for formatting the converted currency result.
Define the CurrencyConverterApp
class
public class CurrencyConverterApp extends JFrame {
- We create a class called
CurrencyConverterApp
that extendsJFrame
, making it a Swing GUI application.
Declare instance variables for GUI components:
private JLabel titleLabel;
private JLabel amountLabel;
private JTextField amountTextField;
private JLabel fromLabel;
private JComboBox<String> fromComboBox;
private JLabel toLabel;
private JComboBox<String> toComboBox;
private JButton convertButton;
private JLabel resultLabel;
private JLabel noteLabel;
- These instance variables represent various GUI components like labels, text fields, combo boxes, buttons, and result labels.
Define arrays for currencies and conversion rates:
private static final String[] currencies = {"USD", "EUR", "GBP", "JPY", "CAD", "INR"};
private static final double[][] conversionRates = {
// Conversion rates from the base currency (USD) to other currencies
{1.0, 0.84, 0.74, 110.94, 1.26, 74.0}, // USD
{1.19, 1.0, 0.88, 131.84, 1.5, 88.29}, // EUR
{1.35, 1.13, 1.0, 149.96, 1.71, 100.53}, // GBP
{0.0090, 0.0076, 0.0067, 1.0, 0.011, 0.65}, // JPY
{0.79, 0.67, 0.59, 88.97, 1.0, 58.64}, // CAD
{0.0135, 0.0113, 0.0099, 1.52, 0.017, 1.0} // INR
};
currencies
is an array of currency codes.conversionRates
is a 2D array representing conversion rates between different currencies.
Create the constructor for the CurrencyConverterApp
class:
public CurrencyConverterApp() {
- This constructor sets up the GUI components and initializes the application.
Set the JFrame title:
setTitle("Currency Converter - Made by updateGadh.com");
- Sets the title of the JFrame window.
Create and configure the titleLabel
:
titleLabel = new JLabel("Currency Converter");
titleLabel.setFont(new Font("Arial", Font.BOLD, 18));
- Creates a label with the text “Currency Converter” and sets its font to bold.
Create and configure other GUI components (e.g., amountLabel
, amountTextField
, fromLabel
, fromComboBox
, etc.):
amountLabel = new JLabel("Enter Amount:");
amountTextField = new JTextField(10);
fromLabel = new JLabel("From Currency:");
fromComboBox = new JComboBox<>(currencies);
toLabel = new JLabel("To Currency:");
toComboBox = new JComboBox<>(currencies);
convertButton = new JButton("Convert");
- These lines create and configure various GUI components for the currency converter form.
Add action listener to the convertButton
:
convertButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
convertCurrency();
}
});
- Sets up an action listener for the “Convert” button. When clicked, it will call the
convertCurrency
method.
Output :-
Source Code:-
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.DecimalFormat;
public class CurrencyConverterApp extends JFrame {
private JLabel titleLabel;
private JLabel amountLabel;
private JTextField amountTextField;
private JLabel fromLabel;
private JComboBox<String> fromComboBox;
private JLabel toLabel;
private JComboBox<String> toComboBox;
private JButton convertButton;
private JLabel resultLabel;
private JLabel noteLabel;
private static final String[] currencies = {"USD", "EUR", "GBP", "JPY", "CAD", "INR"};
private static final double[][] conversionRates = {
{1.0, 0.84, 0.84, 110.94, 1.26, 74.0}, // USD
{1.19, 1.0, 0.88, 131.84, 1.5, 88.29}, // EUR
{1.35, 1.13, 1.0, 149.96, 1.71, 100.53}, // GBP
{0.0090, 0.0076, 0.0067, 1.0, 0.011, 0.65}, // JPY
{0.79, 0.67, 0.59, 88.97, 1.0, 58.64}, // CAD
{0.0135, 0.0113, 0.0099, 1.52, 0.017, 1.0} // INR
};
public CurrencyConverterApp() {
// Set the title of the JFrame
setTitle("Currency Converter - Made by updateGadh.com");
// Create and configure components
titleLabel = new JLabel("Currency Converter");
titleLabel.setFont(new Font("Arial", Font.BOLD, 18));
amountLabel = new JLabel("Enter Amount:");
amountTextField = new JTextField(10);
fromLabel = new JLabel("From Currency:");
fromComboBox = new JComboBox<>(currencies);
toLabel = new JLabel("To Currency:");
toComboBox = new JComboBox<>(currencies);
convertButton = new JButton("Convert");
convertButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
convertCurrency();
}
});
resultLabel = new JLabel("Result: ");
// Add a note label
noteLabel = new JLabel("Note: The currency values used in this demo are for illustration purposes only.");
// Create and configure the layout
setLayout(new FlowLayout());
// Add components to the JFrame
add(titleLabel);
add(amountLabel);
add(amountTextField);
add(fromLabel);
add(fromComboBox);
add(toLabel);
add(toComboBox);
add(convertButton);
add(resultLabel);
add(noteLabel);
// Set default values
amountTextField.setText("100"); // Default amount
fromComboBox.setSelectedItem("USD"); // Default 'From' currency
toComboBox.setSelectedItem("EUR"); // Default 'To' currency
// Set JFrame properties
setSize(400, 250);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null); // Center the JFrame on the screen
setVisible(true);
}
private void convertCurrency() {
try {
double amount = Double.parseDouble(amountTextField.getText());
String fromCurrency = (String) fromComboBox.getSelectedItem();
String toCurrency = (String) toComboBox.getSelectedItem();
// Find the index of 'fromCurrency' and 'toCurrency' in the 'currencies' array
int fromIndex = -1;
int toIndex = -1;
for (int i = 0; i < currencies.length; i++) {
if (currencies[i].equals(fromCurrency)) {
fromIndex = i;
}
if (currencies[i].equals(toCurrency)) {
toIndex = i;
}
}
if (fromIndex != -1 && toIndex != -1) {
double conversionRate = conversionRates[fromIndex][toIndex];
double convertedAmount = amount * conversionRate;
DecimalFormat df = new DecimalFormat("#.##");
resultLabel.setText("Result: " + df.format(amount) + " " + fromCurrency + " = " + df.format(convertedAmount) + " " + toCurrency);
} else {
JOptionPane.showMessageDialog(this, "Invalid currency selection.", "Error", JOptionPane.ERROR_MESSAGE);
}
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(this, "Invalid input. Please enter a valid number.", "Error", JOptionPane.ERROR_MESSAGE);
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new CurrencyConverterApp();
}
});
}
}
1 comment