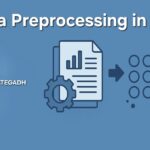
Python sys Module : A Comprehensive Guide
Python sys Module
Python’s sys
module is a powerful utility that provides access to a wide range of system-specific parameters and functions. By tapping into the sys
module, developers can interact directly with the Python runtime environment, making it possible to manage system behavior, access command-line arguments, and interact with essential system parameters. Here, we’ll dive into key features of the sys
module that can streamline your development workflow and enhance your understanding of Python’s runtime behavior.
Getting Started: Importing the sys
Module
You must import the sys module into your script before you may use any of its features:
import sys
Once imported, you’re ready to access its various functions and variables to interact with Python’s runtime.
Download New Real Time Projects :-Click here
Important Variables and Functions
sys.modules
All of the modules that are currently imported into the Python environment are listed in the sys.modules
dictionary. This can be particularly helpful for tracking dependencies and managing module imports dynamically.
import sys
print(sys.modules)
sys.argv
Sys.argv,
one of the most used characteristics, records a list of command-line arguments that are supplied to the script when it is executed. The script’s name appears in the first element, sys.argv[0],
and the following elements stand for more arguments.
import sys
print(sys.argv) # Outputs command-line arguments passed to the script
sys.base_exec_prefix
sys.base_exec_prefix
provides the base directory of the current Python installation. This is often useful for determining the installation environment, especially when working outside a virtual environment.
sys.base_prefix
Similar to sys.base_exec_prefix
, the sys.base_prefix
is set during Python’s startup. It typically points to the Python installation directory and can be helpful for tracking the Python runtime location in different environments.
sys.byteorder
This attribute returns the native byte order (either “little” or “big”) of your system, which is crucial for applications that handle data serialization and binary data operations.
import sys
print(sys.byteorder) # Outputs the byte order of the system
sys.maxsize
The maximum size of a Python integer on the current platform is provided by sys.maxsize.
Knowing this value can be essential when working with large data sets or computations requiring extensive memory.
import sys
print(sys.maxsize) # Outputs the maximum integer size supported
sys.path
sys.path
is a list that includes the directories Python searches for modules. It is a dynamic representation of your PYTHONPATH
environment variable, allowing you to manipulate the search path for modules at runtime.
import sys
print(sys.path) # Displays the current search path for Python modules
https://updategadh.com/category/php-project
sys.stdin
The sys.stdin
object represents the standard input stream and is typically used for reading input in Python scripts. By interacting with sys.stdin
, you can customize input handling or even redirect input from files.
import sys
user_input = sys.stdin.read() # Reads from standard input
sys.getrefcount
The reference count of an object is returned by sys.getrefcount
. This feature is particularly helpful in memory management and debugging memory leaks, as it shows how many references are pointing to an object in memory.
import sys
sample_obj = "Hello, World!"
print(sys.getrefcount(sample_obj)) # Returns reference count of the object
sys.exit
sys.exit
is used to terminate a Python program gracefully. This function is highly useful for closing a script after a certain condition or handling exceptions to prevent further execution.
import sys
sys.exit("Exiting program") # Exits the program and outputs a message
sys.executable
The absolute path to the Python interpreter that is executing the script at the moment is provided by this attribute. It’s invaluable for determining Python’s location, especially in complex environments where multiple versions of Python might be installed.
import sys
print(sys.executable) # Outputs the path to the Python interpreter
sys.platform
sys.platform
is a string that identifies the platform on which the script is running, such as "win32"
for Windows or "linux"
for Linux. This is essential for developing cross-platform applications, as it helps you adjust code behavior based on the system.
import sys
print(sys.platform) # Outputs the platform type
- how to install sys module in python
- sys module in python w3schools
- sys module in python example
- import sys in python
- Python sys Module : A Comprehensive Guide
- how will you display the info on float datatype by importing sys module?
- write the code to display the python version by importing sys module.
- sys.exit python
- Python sys Module
- sys.stdin python
- python os module
- python
- python sys module w3schools
- how will you display the info on float datatype by importing sys module
- write the code to display the python version by importing sys module
- Python sys Module : A Comprehensive Guide
- Python sys Module
Post Comment