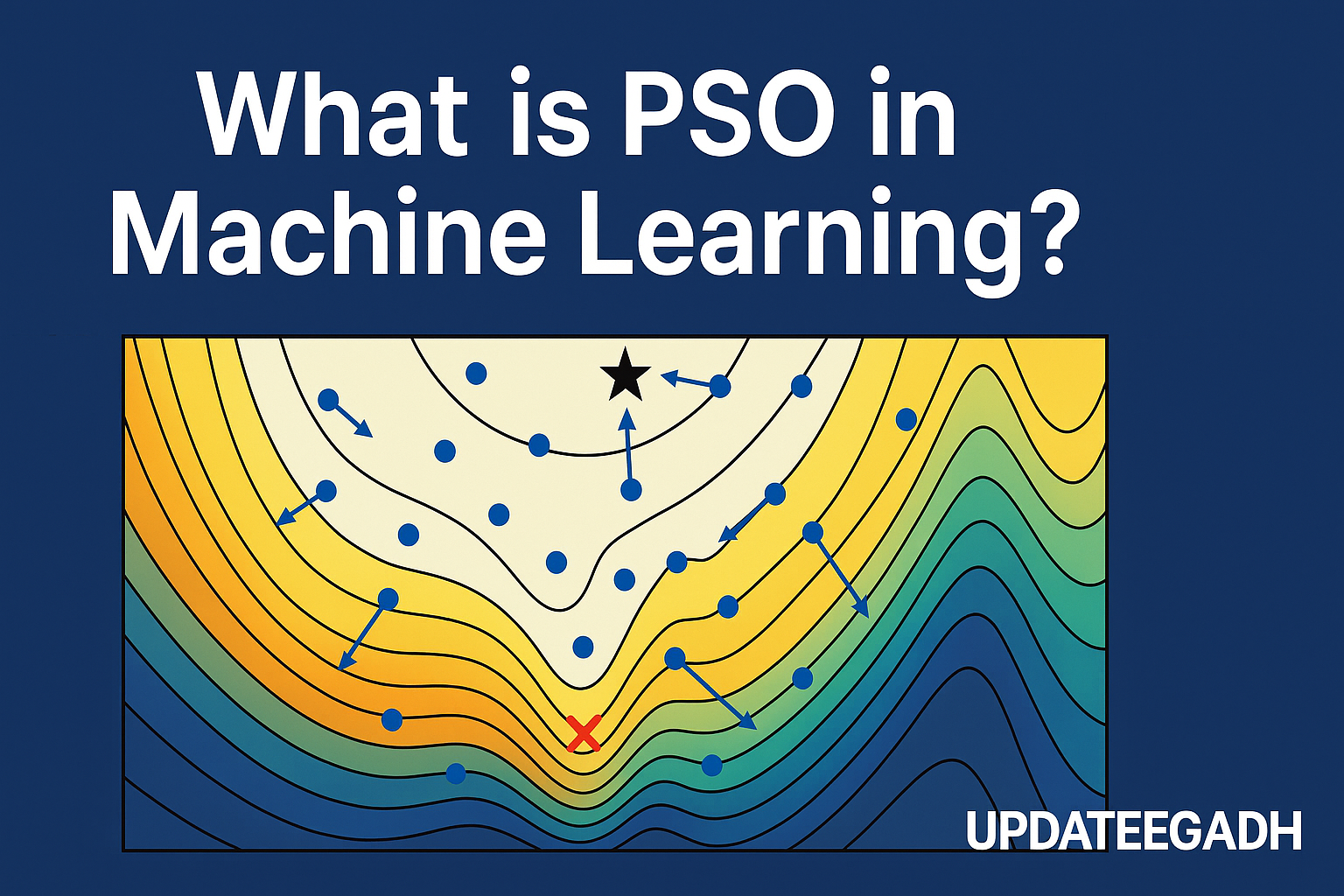
Bus Ticket Booking PHP Free Source Code
The Bus Ticket Booking PHP with Source Code
In today’s fast-paced world, convenience is everything. Whether it’s ordering food, shopping online, or booking travel tickets, people prefer doing it all from the comfort of their smartphones. This shift towards mobile convenience has made bus ticket booking apps increasingly popular. Imagine being able to create such an app—one that allows users to book their bus tickets on the go, complete with an admin panel for seamless management. This article will guide you through the development of a bus ticket booking app for Android, alongside an admin panel built with PHP, providing insight into the source code that makes it all possible.
Understanding the Bus Ticket Booking App
Importance of an Admin Panel
While the user-facing app is crucial for customer interaction, the admin panel is the backbone of the entire system. The admin panel allows bus operators and administrators to manage routes, schedules, seat availability, and bookings. It also handles customer inquiries, monitors system performance, and generates reports. Essentially, the admin panel is where all the behind-the-scenes magic happens, ensuring that the bus ticket booking process runs smoothly for both users and operators.
Core Features
User Features
- User Registration and Authentication: Allows users to create an account, log in, and manage their profiles securely.
- Search for Buses: Users can search for available buses based on their departure and destination points, travel dates, and preferred times.
- Seat Selection: Provides a visual representation of available seats, allowing users to choose their preferred seats.
- Booking and Payment: Users can book their selected seats and make payments through integrated payment gateways.
- Digital Tickets: Once booked, users receive a digital ticket within the app, which they can show when boarding.
- Booking History: Users can view their past bookings, making it easier to rebook or check previous travel details.
- Notifications: The app sends push notifications for booking confirmations, reminders, and any changes in schedules.
Admin Panel Features
- Route Management: Administrators can add, edit, or remove bus routes and schedules.
- Seat Availability Management: Allows real-time updates of seat availability based on bookings and cancellations.
- User Management: Admins can manage user accounts, resolve issues, and handle inquiries.
- Booking Management: View and manage all bookings, including cancellations and refunds.
- Payment Monitoring: Track all transactions, ensuring that payments are processed correctly and securely.
- Reporting and Analytics: Generate reports on bookings, revenue, and system performance to make informed business decisions.
Step-by-Step Guide
1. Setting Up the Development Environment
To start developing the Android app, you need to set up your development environment:
- Android Studio: Download and install Android Studio, which is the official IDE for Android development. It comes with all the tools you need to build and test your app.
- Java/Kotlin: Choose either Java or Kotlin as your programming language. Both are supported in Android development, with Kotlin being the more modern and preferred choice by Google.
2. Designing the User Interface (UI)
The user interface is the first thing users interact with, so it needs to be intuitive and user-friendly.
- Login and Registration Screens: Create screens for user registration and login, including options for social media logins.
// Sample code for a login activity in Java
public class LoginActivity extends AppCompatActivity {
private EditText email, password;
private Button loginButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
email = findViewById(R.id.email);
password = findViewById(R.id.password);
loginButton = findViewById(R.id.login_button);
loginButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String userEmail = email.getText().toString().trim();
String userPassword = password.getText().toString().trim();
// Perform login authentication here
}
});
}
}
- Bus Search and Selection Screens: Design screens that allow users to search for buses, view available options, and select their seats.
- Booking and Payment Screens: Create forms where users can enter booking details and process payments.
3. Implementing the Core Functionalities
- User Authentication: Implement secure login and registration processes, using Firebase Authentication or your custom API.
FirebaseAuth mAuth = FirebaseAuth.getInstance();
mAuth.signInWithEmailAndPassword(email, password)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (task.isSuccessful()) {
// Sign in success
FirebaseUser user = mAuth.getCurrentUser();
// Navigate to home screen
} else {
// If sign in fails, display a message to the user.
}
}
});
- Bus Search and Booking: Integrate an API or backend system that allows users to search for buses, view details, and book seats.
// Pseudo-code for searching buses
public List<Bus> searchBuses(String departure, String destination, String date) {
List<Bus> availableBuses = new ArrayList<>();
// Query the database or API for available buses
return availableBuses;
}
- Payment Gateway Integration: Integrate with payment gateways like PayPal, Stripe, or local providers to process payments securely.
- Notifications: Implement push notifications to alert users about booking confirmations, reminders, and schedule changes.
4. Testing the App
Before releasing your app, thoroughly test it to ensure all functionalities work as expected. Use Android’s built-in testing tools and frameworks like Espresso for UI testing and JUnit for unit testing.
Building the Admin Panel in PHP: A Step-by-Step Guide
1. Setting Up the Development Environment
For the admin panel, you need to set up a PHP development environment:
- XAMPP/WAMP: Install XAMPP or WAMP, which provides a local server environment with Apache, MySQL, and PHP.
- PHPMyAdmin: Use PHPMyAdmin for managing the MySQL database, which will store all the app’s data.
2. Designing the Admin Interface
The admin panel should be intuitive and easy to navigate, allowing administrators to manage operations efficiently.
- Dashboard: Create a dashboard that provides a quick overview of the system’s status, including the number of active users, upcoming trips, and recent bookings.
<?php
// Sample code for an admin dashboard in PHP
session_start();
if (!isset($_SESSION['admin_logged_in'])) {
header("Location: login.php");
exit();
}
?>
<h1>Welcome to the Admin Dashboard</h1>
<p>Total Users: <?php echo $totalUsers; ?></p>
<p>Total Bookings: <?php echo $totalBookings; ?></p>
- Management Sections: Create sections for managing routes, buses, bookings, and users, with forms to add, edit, or delete entries.
3. Implementing Core Functionalities
- Route Management: Allow admins to add, edit, and delete bus routes and schedules, updating the database in real-time.
// Pseudo-code for adding a new bus route
if (isset($_POST['add_route'])) {
$departure = $_POST['departure'];
$destination = $_POST['destination'];
$time = $_POST['time'];
$query = "INSERT INTO routes (departure, destination, time) VALUES ('$departure', '$destination', '$time')";
mysqli_query($connection, $query);
}
- Booking Management: Enable admins to view all bookings, process cancellations, and issue refunds as needed.
// Pseudo-code for viewing all bookings
$query = "SELECT * FROM bookings ORDER BY date DESC";
$result = mysqli_query($connection, $query);
while ($booking = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $booking['id'] . "</td>";
echo "<td>" . $booking['user_id'] . "</td>";
echo "<td>" . $booking['route_id'] . "</td>";
echo "<td>" . $booking['date'] . "</td>";
echo "<td>" . $booking['status
'] . "</td>";
echo "</tr>";
}
Example output:
Console Output:
- User Management: Provide functionalities to view and manage user accounts, handle customer support issues, and monitor system usage.
- Reporting: Implement features for generating reports on bookings, revenue, and other key metrics.
4. Testing the Admin Panel
Just like the app, the admin panel needs thorough testing to ensure it handles all tasks correctly. Test for usability, security, and data integrity, ensuring that the system is robust and reliable.
The Importance of Source Code
The source code is where the entire system’s functionality is defined. Having access to the source code means you can customize the app and admin panel to meet specific business needs, fix bugs, and continuously improve the system as user requirements evolve. It’s the foundation upon which you build a reliable, efficient, and user-friendly bus ticket booking service.
- New Project :-https://www.youtube.com/@Decodeit2
- PHP PROJECT:- CLICK HERE
- Bus Ticket Booking PHP with Source Code
- Bus Ticket Booking PHP
1 comment