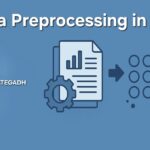
Python SimpleImputer Module: A Comprehensive Guide
Python SimpleImputer Module
Handling missing data is a critical step in data preprocessing for predictive modeling. The SimpleImputer module in Scikit-learn (formerly known as the impute
module) provides an elegant solution to this issue. This tutorial delves into the SimpleImputer class, demonstrating how to replace missing values in datasets with ease using Python.
Table of Contents
- Python SimpleImputer Module
Download New Real Time Projects :-Click here
What is the SimpleImputer Class?
The SimpleImputer class in Scikit-learn is designed to handle missing values in a dataset by imputing them with a specific placeholder value. It replaces missing values (commonly represented as NaN
) with a central tendency measure such as the mean, median, or mode, or even a constant value, depending on your requirements.
Syntax of the SimpleImputer Class
You can use the following syntax to access the SimpleImputer class:
SimpleImputer(missing_values, strategy, fill_value)
Parameters:
missing_values
:
The placeholder for missing values in the dataset. The default isNaN
.strategy
:
Specifies the method to replace missing values. Options include:
- ‘mean’ (default): Uses the column mean to fill in the missing values.
- “median”: Uses the column median to fill in the missing values.
- ‘most_frequent’: Provides the mode in place of missing values.
- “constant”: Provides a constant value in place of missing values.
fill_value
:
Used when thestrategy
is set to'constant'
. The constant value to replace missing data is defined by this parameter.
Installing Scikit-learn
Before utilizing the SimpleImputer class, ensure that the Scikit-learn library is installed. The command below can be used to accomplish this:
pip install sklearn
Once installed, you’re ready to work with the SimpleImputer module.
Handling Missing Data with SimpleImputer
To understand the SimpleImputer class better, let’s explore a practical example where we handle missing values in a dataset.
Example: Replacing Missing Values with the Mean
Here’s how to use the SimpleImputer to substitute the mean for missing values in a dataset:
# Import required modules
import numpy as np
from sklearn.impute import SimpleImputer
# Define the dataset with missing values
dataSet = [
[32, np.nan, 34, 47],
[17, np.nan, 71, 53],
[19, 29, np.nan, 79],
[np.nan, 31, 23, 37],
[19, np.nan, 79, 53]
]
# Print the original dataset
print("Original Dataset:")
print(dataSet)
# Create a SimpleImputer object with strategy 'mean'
imputer = SimpleImputer(missing_values=np.nan, strategy='mean')
# Fit the imputer on the dataset and transform it
imputed_data = imputer.fit_transform(dataSet)
# Print the dataset after imputing missing values
print("\nImputed Dataset:")
print(imputed_data)
Output:
Original Dataset:
[[32, nan, 34, 47], [17, nan, 71, 53], [19, 29, nan, 79], [nan, 31, 23, 37], [19, nan, 79, 53]]
Imputed Dataset:
[[32. 30. 34. 47. ]
[17. 30. 71. 53. ]
[19. 29. 51.75 79. ]
[21.75 31. 23. 37. ]
[19. 30. 79. 53. ]]
Explanation of the Code
- Importing Libraries:
We import thenumpy
library to handle missing values and Scikit-learn’sSimpleImputer
to impute them. - Dataset Creation:
A dataset containing missing values (NaN
) is defined. - SimpleImputer Configuration:
An instance ofSimpleImputer
is created withstrategy='mean'
, indicating that missing values will be replaced by the mean of the corresponding column. - Fitting and Transforming the Data:
The replacement values are computed and applied to the dataset using the fit_transform method. - Output:
The imputed dataset is shown, with the column means used to fill in the missing values.
- PHP PROJECT:- CLICK HERE
- INTERVIEW QUESTION:-CLICK HERE
- Complete Advance AI topics:- CLICK HERE
- Complete Python Course with Advance topics:- CLICK HERE
simpleimputer example
python simpleImputer module
simple imputer for categorical data
sklearn simpleimputer
Python SimpleImputer Module
simpleimputer vs fillna
simpleimputer(strategy)
simpleimputer fit
columntransformer
iterativeimputer
Python SimpleImputer Module
python simpleimputer module missing values
python simpleimputer module w3schools
python simpleimputer module example
Python SimpleImputer Module: A Comprehensive Guide
Post Comment