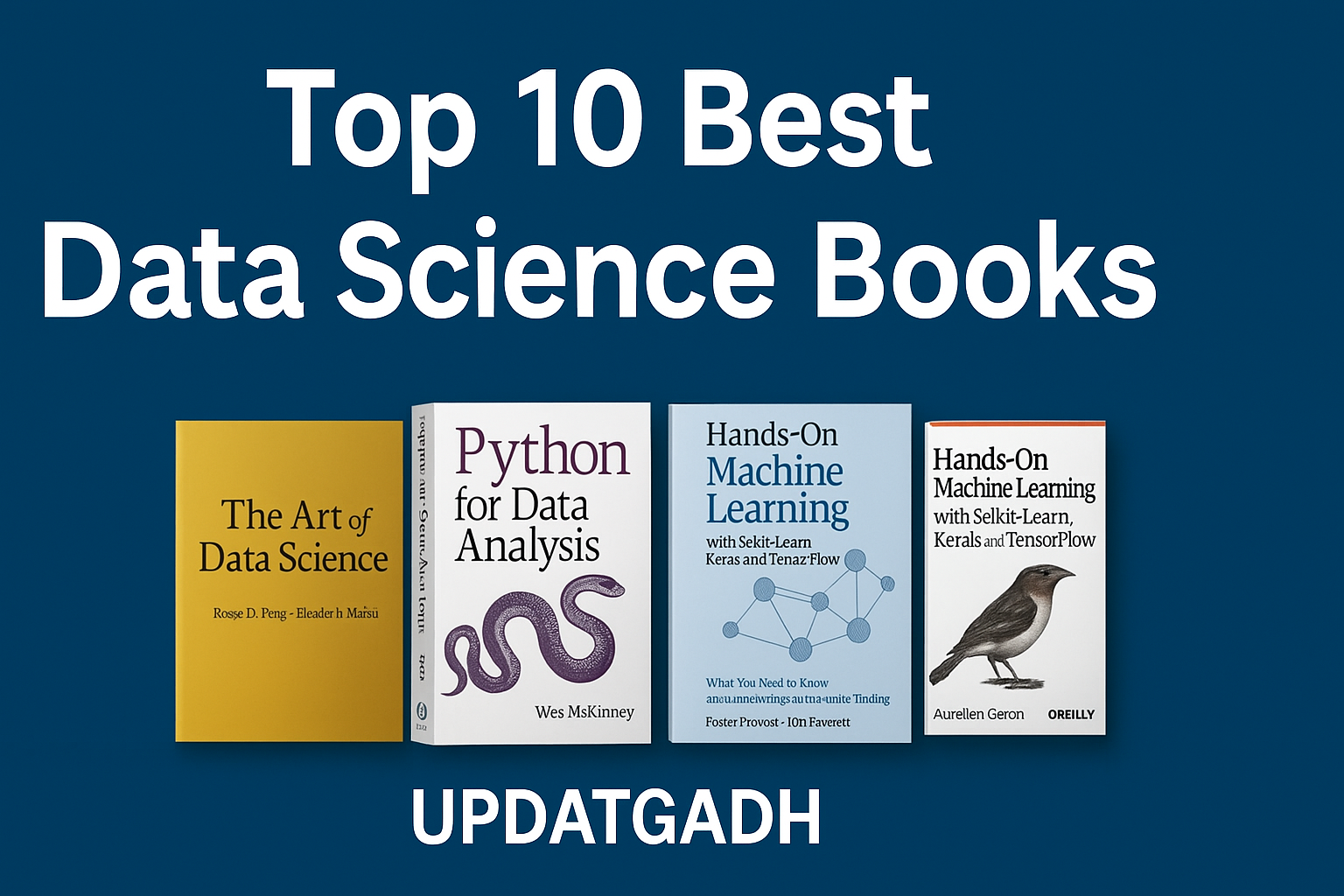
Account Management System in Python Free Source Code
Introduction
Account Management System in Python Free Source Code
In a world where every penny counts and financial decisions can shape our future, the importance of staying organized with your finances cannot be overstated. Managing multiple accounts, tracking expenses, and ensuring that every transaction is recorded accurately can sometimes feel like a daunting task. But imagine having a tool that not only simplifies this process but also empowers you to take control of your financial destiny.
Table of Contents
Understanding
An Account Management System is a software application designed to help you manage your financial accounts. It allows you to create, update, and manage multiple accounts, record transactions, and generate reports, all in one place. The system’s primary goal is to give you control over your finances, ensuring that every detail is accounted for and easily accessible when needed.
Core Features
- User Authentication: Secure login and registration to ensure that only authorized users can access the system.
- Account Creation and Management: The ability to create new accounts, update account details, and delete accounts as needed.
- Transaction Management: Recording and managing income and expenses, along with detailed transaction histories.
- Reporting: Generating reports that provide insights into account balances, spending patterns, and overall financial health.
These features work together to provide a comprehensive solution for managing your finances, making it easier to stay organized and make informed decisions.
Step-by-Step Guide
1. Setting Up Your Environment
Before you begin coding, make sure you have Python installed on your system. You’ll also need an Integrated Development Environment (IDE) like PyCharm or VS Code.
pip install sqlite3
2. Designing the Database
We’ll use SQLite, a lightweight and easy-to-use database, to store account information and transactions.
import sqlite3
def create_database():
conn = sqlite3.connect('account_management.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS accounts
(id INTEGER PRIMARY KEY, name TEXT, balance REAL)''')
c.execute('''CREATE TABLE IF NOT EXISTS transactions
(id INTEGER PRIMARY KEY, account_id INTEGER, amount REAL,
type TEXT, date TEXT, FOREIGN KEY(account_id) REFERENCES accounts(id))''')
conn.commit()
conn.close()
create_database()
3. Implementing Core Functions
Create a New Account:
def create_account(name, initial_balance):
conn = sqlite3.connect('account_management.db')
c = conn.cursor()
c.execute("INSERT INTO accounts (name, balance) VALUES (?, ?)", (name, initial_balance))
conn.commit()
conn.close()
create_account('Savings', 1000.00)
Record a Transaction:
from datetime import datetime
def record_transaction(account_id, amount, transaction_type):
conn = sqlite3.connect('account_management.db')
c = conn.cursor()
date = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
c.execute("INSERT INTO transactions (account_id, amount, type, date) VALUES (?, ?, ?, ?)",
(account_id, amount, transaction_type, date))
# Update account balance
if transaction_type == 'deposit':
c.execute("UPDATE accounts SET balance = balance + ? WHERE id = ?", (amount, account_id))
elif transaction_type == 'withdrawal':
c.execute("UPDATE accounts SET balance = balance - ? WHERE id = ?", (amount, account_id))
conn.commit()
conn.close()
record_transaction(1, 500.00, 'deposit')
Generate a Report:
def generate_report(account_id):
conn = sqlite3.connect('account_management.db')
c = conn.cursor()
c.execute("SELECT * FROM transactions WHERE account_id = ?", (account_id,))
transactions = c.fetchall()
conn.close()
print(f"Transaction Report for Account ID: {account_id}")
for transaction in transactions:
print(transaction)
generate_report(1)
The Emotional Impact of Financial Organization
There’s something incredibly empowering about having control over your finances. When you have a system that tracks every dollar, records every transaction, and provides you with a clear picture of your financial health, you’re not just managing money—you’re managing your future.
Conclusion
Building an Account Management System in Python is more than just a programming exercise; it’s an investment in your financial well-being. With this system, you can take control of your accounts, gain insights into your spending habits, and make informed decisions that will benefit you in the long run.
- New Project :-https://www.youtube.com/@Decodeit2
- PHP PROJECT:- CLICK HERE
- what is account management system
- what is account management
- what is user account management
- library management system in python
- what is bank account management
- how to use python in accounting
- python accounting
- bank account python project
- Account Management System in Python With Source Code
- Account Management System in Python
- Account Management System in Python Free Source Code
Post Comment