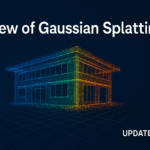
Logging in Python: A Comprehensive Guide
Logging in Python
Introduction
Logging is an essential aspect of software development that allows developers to track and record events that occur during program execution. Python’s built-in logging
module provides a robust framework for logging messages at different severity levels, helping developers diagnose issues efficiently.
In this tutorial, we will explore the fundamentals of the logging
module, including its features, logging levels, basic configurations, formatting, and advanced usage.
Complete Python Course with Advance topics:-Click here
What is Logging?
Logging in Python is a mechanism for tracking events that happen when a program runs. It helps developers understand what the program is doing and aids in debugging. Instead of using print statements for debugging, logging provides a structured and configurable way to record messages.
Why Use Logging?
- Diagnosing Problems: Helps trace the cause of errors or unexpected behaviors.
- Record-Keeping: Maintains logs for auditing and monitoring purposes.
- Debugging: Provides more context than simple print statements.
- Error Handling: Helps in identifying and handling runtime issues.
How Logging Works
The logging
module provides five levels of logging severity:
Level | Numeric Value | Description |
---|---|---|
DEBUG |
10 | Detailed debugging information. |
INFO |
20 | General events, confirming that things are working as expected. |
WARNING |
30 | An indication of something unexpected that might cause issues later. |
ERROR |
40 | A serious problem that prevents the program from executing a specific task. |
CRITICAL |
50 | A severe error that might cause the program to stop running entirely. |
Example:
import logging
logging.debug("This is a debug message")
logging.info("This is an info message")
logging.warning("This is a warning message")
logging.error("This is an error message")
logging.critical("This is a critical message")
Output:
WARNING:root:This is a warning message
ERROR:root:This is an error message
CRITICAL:root:This is a critical message
Explanation:
- By default, Python logs messages at
WARNING
level and above. DEBUG
andINFO
messages are ignored unless explicitly configured.
Configuring Logging
To change the default behavior, we can use basicConfig()
to specify the logging level, format, and output destination.
Setting the Logging Level
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug("This debug message will be displayed now")
Output:
DEBUG:root:This debug message will be displayed now
Logging to a File
Instead of printing logs to the console, we can save them to a file.
import logging
logging.basicConfig(filename='app.log', filemode='w', level=logging.WARNING)
logging.warning("This warning will be logged in the file")
This creates a file app.log
with the logged message.
Custom Formatting
We can format log messages to include additional details like timestamp, function name, and log level.
import logging
logging.basicConfig(format='%(asctime)s - %(levelname)s - %(message)s',
level=logging.INFO)
logging.info("User logged in")
Output:
2025-02-11 10:30:45,123 - INFO - User logged in
Setting Date and Time Format
import logging
logging.basicConfig(format='%(asctime)s - %(message)s', datefmt='%d-%b-%y %H:%M:%S')
logging.warning("Database connection failed")
Output:
11-Feb-25 10:45:30 - Database connection failed
Using Logger Objects
Instead of using the root logger, we can create custom loggers for better control.
import logging
# Create logger
logger = logging.getLogger("CustomLogger")
logger.setLevel(logging.DEBUG)
# Create handler
handler = logging.FileHandler("custom.log")
formatter = logging.Formatter('%(name)s - %(levelname)s - %(message)s')
handler.setFormatter(formatter)
logger.addHandler(handler)
# Logging messages
logger.debug("Debugging the application")
logger.info("Application started")
This setup allows better organization and log management, especially for large applications.
Capturing Stack Traces
We can log stack traces for exceptions using exception()
, which automatically includes traceback information.
import logging
logging.basicConfig(level=logging.ERROR)
try:
result = 10 / 0
except ZeroDivisionError:
logging.exception("An error occurred")
Output:
ERROR:root:An error occurred
Traceback (most recent call last):
File "example.py", line 5, in <module>
result = 10 / 0
ZeroDivisionError: division by zero
Logging Variable Data
We can pass dynamic data into log messages using string formatting.
import logging
user = "Alice"
logging.error("%s attempted an unauthorized action", user)
Or using f-strings (Python 3.6+):
logging.error(f"{user} attempted an unauthorized action")
Download New Real Time Projects :-Click here
Complete Advance AI topics:- CLICK HERE
Conclusion
Logging in Python is a powerful tool that helps developers track and troubleshoot issues effectively. By understanding different log levels, configurations, and advanced features like loggers, handlers, and formatting, developers can enhance their debugging experience and maintain cleaner code.
If you want to dive deeper, refer to Python’s official documentation: Python Logging Module.
Logging in Python
Logging in Python example
Logging in Python to file
python logging to console
logger.info python
Logging in Python to stdout
python logging best practices
Logging in Python levels
python logging not printing
online python compiler
logging in python w3schools
Post Comment