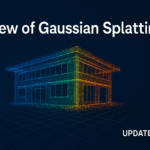
How to Read a Text File in Python
How to Read a Text File in Python
Python makes handling files seamless and straightforward. Whether you want to read, write, or create files, Python offers robust tools to work with both text and binary files.
Let’s dive into understanding these file types and explore how to read a text file in Python step-by-step.
Complete Advance AI topics:- CLICK HERE
Complete Python Course with Advance topics:-Click here
Types of Files in Python
- Text Files:
Text files store characters in plain text, terminated by a special character called EOL (End of Line). By default, Python uses\n
to denote a new line in text files. - Binary Files:
Binary files store data in binary format (a sequence of 1s and 0s). Unlike text files, binary files do not use EOL terminators.
In this article, we will focus on reading text files using Python.
Steps to Read a Text File in Python
Python follows three essential steps when working with text files:
- Open a file
- Read or write to the file
- Close the file
1. Opening a Text File
To open a file, Python provides the built-in open()
function. This function returns a file object that can be used to perform various operations like reading, writing, or appending.
Syntax:
file_object = open(file_name, mode)
file_name
: The name (or path) of the file to open.mode
: Specifies the mode in which the file is opened. Common modes include:r
: Read mode (default)w
: Write modea
: Append mode
Example:
file_path = "example.txt"
file = open(file_path, "r") # Open file in read mode
Here, file
is the file object, and "r"
specifies that the file is being opened for reading.
2. Reading a Text File
Python offers several methods to read the content of a file, but the most commonly used is the read()
function.
Using read()
Function
The read()
function reads the file content. You can optionally pass the number of characters to read as an argument. If no size is provided, the entire file is read.
Example:
file_information = file.read() # Read the entire file content
print(file_information)
This reads the content of the file into the variable file_information
and prints it.
3. Closing a File
Once you’ve performed the required operations, it’s good practice to close the file using the close()
method. This releases the system resources associated with the file.
Example:
file.close()
Complete Example Program
Here’s a complete example demonstrating how to read a text file in Python, including reading specific characters and closing the file:
# Open the file in read mode
file = open(r"C:\Users\Example\example.txt", 'r')
# Read the first 4 characters
content_part1 = file.read(4)
print("First 4 characters:", content_part1)
# Read the next 10 characters
content_part2 = file.read(10)
print("Next 10 characters:", content_part2)
# Read the rest of the file
remaining_content = file.read()
print("Remaining file content:")
print(remaining_content)
# Close the file
file.close()
Output:
First 4 characters: This
Next 10 characters: is line 1
Remaining file content:
This is line 2
This is line 3
This is line 4
Explanation of Code
- Opening the File: The file is opened in read mode using
open()
. Ther
mode ensures no changes are made to the file content. - Reading Characters: The
read()
function reads a specific number of characters when a size is specified (e.g., 4, 10). - Reading the Remaining File: Without arguments,
read()
fetches the remaining content. - Closing the File: The
close()
function ensures the file is properly closed, freeing system resources.
Updated Practices
Instead of manually opening and closing files, Python provides a more efficient way to handle files using a with
statement. This approach ensures the file is automatically closed after operations are completed.
Example with with
Statement:
file_path = "example.txt"
with open(file_path, "r") as file:
content = file.read()
print(content) # Automatically closes the file after reading
Download New Real Time Projects :-Click here
how to read a text file in python using pandas
python read text file line by line
how to read a text file in python example
how to read a text file in python w3schools
how to read a text file in python
how to read a text file in python using pandas
how to read a text file in python using numpy
how to read a text file in python without newline
how to read a text file in python stack overflow
how to read a text file in python with open
how to read all text file in python
how to read and write text file in python
Post Comment