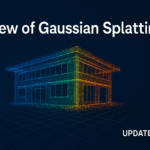
How to Convert Text to Speech in Python
How to Convert Text to Speech in Python
In this tutorial, we will learn how to convert human language text into human-like speech using Python.
Sometimes, we prefer listening to content instead of reading. Listening allows multitasking, making it easier to absorb critical information while performing other tasks. Google Text-to-Speech (gTTS) is one of the most widely used Python text-to-speech APIs available.
The gTTS API is easy to use and comes with built-in functions that allow us to save text as an MP3 file. Unlike complex neural network models that require extensive training, gTTS simplifies text-to-speech conversion.
Complete Python Course with Advance topics:-Click here
Features of gTTS API
- supports a number of languages, such as French, Tamil, German, Hindi, and English.
- Allows speech playback in slow or normal speed modes.
- Simple and lightweight solution for converting text into audio.
However, one limitation of gTTS is that the generated speech file cannot be modified once created. To overcome this, we will also explore an offline library called pyttsx3
that provides more control over the generated speech.
Installing Required Libraries
Installing the required Python libraries is the first step.
pip install gTTS
pip install playsound
pip install pyttsx3
Using gTTS for Text-to-Speech Conversion
Let’s look at a basic example to learn how to utilize the gTTS API.
from gtts import gTTS # Import Google Text-to-Speech API
from playsound import playsound # Import playsound for playing audio
# Define the text to be converted into speech
text_val = "Welcome to UpdateGadh"
# Define language
language = 'en'
# Convert text to speech
speech_obj = gTTS(text=text_val, lang=language, slow=False)
# Save the converted speech as an audio file
speech_obj.save("welcome.mp3")
# Play the saved audio file
playsound("welcome.mp3")
Output:
The script will generate an MP3 file and play the audio output saying, “Welcome to UpdateGadh.”
Getting the List of Supported Languages
To get the list of available languages in gTTS, use the following code:
from gtts.lang import tts_langs
# Fetch supported languages
gtts_languages = tts_langs()
print(gtts_languages)
This will output a dictionary of supported languages with their respective language codes.
Offline Text-to-Speech Conversion with pyttsx3
If you prefer an offline solution, pyttsx3
is a great alternative. It works with pre-installed TTS engines on your system and provides greater control over speech properties such as speed and voice selection.
Example:
import pyttsx3 # Import pyttsx3 for offline TTS
# Initialize the TTS engine
engine = pyttsx3.init()
# Define the text to be spoken
text = "Python is a powerful programming language"
# Convert text to speech
engine.say(text)
# Play the converted speech
engine.runAndWait()
Adjusting Speech Properties
1. Changing Speech Speed:
engine.setProperty("rate", 150) # Set speech rate
2. Changing Voice:
voices = engine.getProperty("voices")
engine.setProperty("voice", voices[1].id) # Change voice
Download New Real Time Projects :-Click here
Complete Advance AI topics:- CLICK HERE
Conclusion
In this lesson, we looked at using Python to turn text into speech. We discussed offline (pyttsx3) and online (gTTS) methods. The gTTS API provides a quick and easy way to generate speech but requires an internet connection, whereas pyttsx3 allows offline conversion with greater control over voice parameters.
With these tools, you can create text-to-speech applications, virtual assistants, or accessibility solutions with ease. Keep experimenting and exploring new ways to integrate TTS into your projects!
how to convert text to speech in python offline
text to speech converter project in python with source code
text-to-speech python code github
How to Convert Text to Speech in Python gtts
How to Convert Text to Speech in Python
how to convert text to speech in python
how to convert text to speech in python offline
How to Convert Text to Speech in Python text-to-speech python pyttsx3
python speech to text real-time
google text-to-speech python
speech recognition python
Post Comment