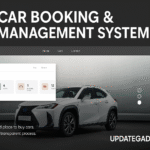
How to Clear the Python Shell
How to Clear the Python Shell
When working with the Python shell, we often encounter cluttered output or unnecessary statements. In such cases, we may want to clear the screen for a fresh start. While clearing a terminal (command prompt or terminal window) is straightforward, it’s a bit different when using Python’s IDLE shell.
Complete Python Course with Advance topics:-Click here
Clearing the Terminal in Windows and Linux
For clearing the terminal in Windows and Linux, we can use the following commands:
- For Windows:
import os os.system('cls')
- For Linux/macOS:
import os os.system('clear')
These commands execute the system command to clear the terminal. However, if you’re using IDLE, these won’t work because IDLE’s shell does not support terminal commands.
Clearing the Python IDLE Shell
Unfortunately, IDLE does not provide a built-in way to clear the screen. The best alternative is to print multiple new lines to push old content out of view:
print("\n" * 100)
Creating a Custom Function
Instead of writing the print statement every time, we can define a function:
def cls():
print("\n" * 100)
Call this function when needed:
cls()
This approach does not remove previous outputs but pushes them out of sight.
Clearing the Shell Using a Python Script
To clear the terminal dynamically based on the operating system, we can use the os
and time
modules:
# Import necessary modules
from os import system, name
from time import sleep
# Define the clear function
def clear():
if name == 'nt': # Windows
_ = system('cls')
else: # Linux/macOS
_ = system('clear')
# Print sample text multiple times
print('Hello\n' * 10)
# Wait for 2 seconds before clearing the screen
sleep(2)
# Call the function to clear the screen
clear()
Explanation:
- The
system
function runs the appropriate command (cls
orclear
) based on the OS. - The underscore (
_
) variable is used because Python shells store the last output in it, but we do not need the return value ofsystem()
. sleep(2)
pauses the execution for 2 seconds before clearing the screen.
Download New Real Time Projects :-Click here
Complete Advance AI topics:- CLICK HERE
Conclusion
Clearing the Python shell varies depending on the environment. While IDLE does not support direct clearing, using multiple new lines or restarting the shell are alternatives. In terminal-based environments, using os.system('cls')
or os.system('clear')
works effectively. If frequent clearing is needed, defining a custom function simplifies the process.
Stay updated with more Python tips and tricks at updategadh!
how to clear screen in python idle in windows
how to clear the python shell in windows 10
how to clear the python shell in windows 11
how to clear the python terminal in vs code
how to clear the python shell mac
how to clear the python shell
command to clear python shell
how to clear the python shell mac
how to clear the shell in idle
Post Comment