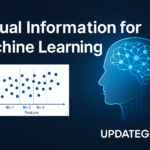
AI-Based Language Translator in Python with Free Code
AI-Based Language Translator in Python
In a world where global communication is key, language barriers can pose significant challenges. Thanks to the rapid advancements in Artificial Intelligence (AI), we now have tools that can break down these barriers by translating languages in real-time. Whether you are traveling abroad, collaborating with an international team, or simply trying to understand content in a foreign language, an AI-based language translator can be your gateway to seamless communication.
In this blog post, we’ll walk through the creation of a simple AI-based language translator using Python, leveraging popular libraries like Googletrans (Google’s translation API). We’ll also provide you with the complete source code to get started.
- Real-World Application: It allows users to translate text between multiple languages, making it a practical and useful tool.
- Enhancing AI Skills: You can learn about AI-based natural language processing (NLP) and machine translation.
- Expandable Features: Start with a basic translator and expand it into a more complex system with additional features like speech translation or multilingual support.
Getting Started: Tools and Libraries
To build our AI-based language translator, we will use Python because of its simplicity and the availability of a vast array of libraries. For this project, we will use:
- Googletrans: A Python library that uses Google’s translation service.
- Tkinter: To create a simple graphical user interface (GUI) for our translator.
Before we dive into the source code, ensure you have these dependencies installed:
pip install googletrans==4.0.0-rc1
pip install tkinter
Source Code:
Here’s the step-by-step guide to building your language translator.
1. Import Required Libraries
from tkinter import *
from googletrans import Translator
- Tkinter will be used to build the user interface, and Translator from Googletrans will help us perform the translations.
PHP PROJECT:- CLICK HERE
2. Create the Main Window
Let’s set up the main window for our translator app using Tkinter.
# Initialize the Tkinter window
root = Tk()
root.title("AI-Based Language Translator")
root.geometry("400x400")
# Heading
Label(root, text="Language Translator", font=("Arial", 18, "bold")).pack(pady=20)
3. Set Up the Input and Output Areas
We’ll create text boxes where users can input text and see the translated output.
# Input Text Box
Label(root, text="Enter Text", font=("Arial", 12)).pack()
input_text = Text(root, height=5, width=40)
input_text.pack(pady=10)
# Output Text Box
Label(root, text="Translated Text", font=("Arial", 12)).pack()
output_text = Text(root, height=5, width=40)
output_text.pack(pady=10)
New Project :-https://www.youtube.com/@Decodeit2
Full Code
from tkinter import *
from googletrans import Translator, LANGUAGES
from tkinter import messagebox
# Initialize the Tkinter window
root = Tk()
root.title("AI-Based Language Translator")
root.geometry("600x400")
# Initialize the Translator
translator = Translator()
# Function to Translate Text
def translate_text():
try:
# Get the input text
text = input_text.get("1.0", "end-1c")
if text == "":
messagebox.showerror("Error", "Please enter some text to translate!")
return
# Get source and destination languages
src_lang = src_lang_var.get()
dest_lang = dest_lang_var.get()
# Perform the translation
translation = translator.translate(text, src=src_lang, dest=dest_lang)
# Display the translated text
output_text.delete("1.0", "end")
output_text.insert("end", translation.text)
except Exception as e:
messagebox.showerror("Translation Error", str(e))
# Heading
Label(root, text="AI-Based Language Translator", font=("Arial", 20, "bold")).pack(pady=10)
# Source Language Dropdown
Label(root, text="Select Source Language", font=("Arial", 12)).pack()
src_lang_var = StringVar(root)
src_lang_var.set("en") # Default source language (English)
# Create a dropdown for source languages
src_lang_menu = OptionMenu(root, src_lang_var, *LANGUAGES.keys())
src_lang_menu.pack(pady=5)
# Input Text Box
Label(root, text="Enter Text", font=("Arial", 12)).pack()
input_text = Text(root, height=5, width=60)
input_text.pack(pady=10)
# Destination Language Dropdown
Label(root, text="Select Destination Language", font=("Arial", 12)).pack()
dest_lang_var = StringVar(root)
dest_lang_var.set("fr") # Default destination language (French)
# Create a dropdown for destination languages
dest_lang_menu = OptionMenu(root, dest_lang_var, *LANGUAGES.keys())
dest_lang_menu.pack(pady=5)
# Output Text Box
Label(root, text="Translated Text", font=("Arial", 12)).pack()
output_text = Text(root, height=5, width=60)
output_text.pack(pady=10)
# Translate Button
Button(root, text="Translate", command=translate_text, font=("Arial", 12), bg="lightblue").pack(pady=20)
# Run the application
root.mainloop()
Key Features:
- Language Selection: Users can select both the source and destination languages from drop-down menus, making the translator more flexible.
- Error Handling: If no text is entered or there’s an issue with translation, a message box displays an error.
- Improved User Interface: The layout is more organized with clear labels for both text input and output.
- AI-Based Language Translator in Python with Source Code
- AI-Based Language Translator in Python
- AI-Based Language Translator in python
Post Comment