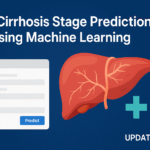
Popular Java Coding Questions & Answer for 2025
Popular Java Coding Questions
Java remains a powerful, adaptable programming language, and it’s no surprise that Java-based questions frequently feature in technical interviews and coding tests. In 2025, Java coding questions focus heavily on practical understanding, problem-solving abilities, and adaptability to real-world challenges. Whether you’re a new developer or brushing up on your Java skills for an upcoming interview, here’s a look at some of the most popular Java coding questions of the year, complete with insights on tackling them successfully.
1.Use No Built-In Functions to Reverse a String
Problem: Write a Java function to reverse a given string without using any built-in functions.
Approach:
This question tests your understanding of basic string manipulation and your problem-solving skills. To solve it, you can use a simple loop to swap characters from the start and end until you meet in the middle.
Sample Solution:
public class StringReversal {
public static String reverseString(String str) {
char[] chars = str.toCharArray();
int left = 0, right = chars.length - 1;
while (left < right) {
char temp = chars[left];
chars[left] = chars[right];
chars[right] = temp;
left++;
right--;
}
return new String(chars);
}
public static void main(String[] args) {
String str = "JavaCode";
System.out.println("Reversed string: " + reverseString(str));
}
}
2. Determine a String’s First Non-Repeated Character
Problem: Determine the first character in a string that doesn’t repeat.
Approach:
This question gauges your proficiency with data structures such as HashMap
. The first non-repeated character can be found by counting how often each character appears.
Sample Solution:
import java.util.HashMap;
public class FirstNonRepeatedCharacter {
public static Character firstNonRepeated(String str) {
HashMap<Character, Integer> charCount = new HashMap<>();
for (char c : str.toCharArray()) {
charCount.put(c, charCount.getOrDefault(c, 0) + 1);
}
for (char c : str.toCharArray()) {
if (charCount.get(c) == 1) {
return c;
}
}
return null;
}
public static void main(String[] args) {
String str = "swiss";
System.out.println("First non-repeated character: " + firstNonRepeated(str));
}
}
Download New Real Time Projects :-Click here
3. Check if a Number is Prime
Problem: Write a Java function that checks if a given number is a prime.
Approach:
Prime-checking is a common interview question, especially for roles requiring efficient algorithms. An optimal solution is to check divisibility up to the square root of the number.
Sample Solution:
public class PrimeCheck {
public static boolean isPrime(int n) {
if (n <= 1) return false;
for (int i = 2; i <= Math.sqrt(n); i++) {
if (n % i == 0) return false;
}
return true;
}
public static void main(String[] args) {
int num = 29;
System.out.println(num + " is prime: " + isPrime(num));
}
}
4. Find the Longest Palindromic Substring
Problem: Given a string, find the longest substring that is a palindrome.
Approach:
This question challenges your understanding of dynamic programming or two-pointer techniques. The goal is to use pointers to expand from the middle of potential palindromic centers.
Sample Solution:
public class LongestPalindrome {
public static String longestPalindrome(String s) {
if (s == null || s.length() < 1) return "";
int start = 0, end = 0;
for (int i = 0; i < s.length(); i++) {
int len1 = expandAroundCenter(s, i, i);
int len2 = expandAroundCenter(s, i, i + 1);
int len = Math.max(len1, len2);
if (len > end - start) {
start = i - (len - 1) / 2;
end = i + len / 2;
}
}
return s.substring(start, end + 1);
}
private static int expandAroundCenter(String s, int left, int right) {
while (left >= 0 && right < s.length() && s.charAt(left) == s.charAt(right)) {
left--;
right++;
}
return right - left - 1;
}
public static void main(String[] args) {
String str = "babad";
System.out.println("Longest palindromic substring: " + longestPalindrome(str));
}
}
https://updategadh.com/category/php-project
5. Implement a Simple LRU Cache
Problem: Implement an LRU (Least Recently Used) Cache with get
and put
methods in Java.
Approach:
This question requires an understanding of caching mechanisms and data structures like HashMap
and LinkedList
. Java’s LinkedHashMap
provides a convenient way to implement LRU caches by maintaining an ordered insertion.
Sample Solution:
import java.util.LinkedHashMap;
import java.util.Map;
public class LRUCache<K, V> extends LinkedHashMap<K, V> {
private final int capacity;
public LRUCache(int capacity) {
super(capacity, 0.75f, true);
this.capacity = capacity;
}
@Override
protected boolean removeEldestEntry(Map.Entry<K, V> eldest) {
return size() > capacity;
}
public static void main(String[] args) {
LRUCache<Integer, String> cache = new LRUCache<>(3);
cache.put(1, "A");
cache.put(2, "B");
cache.put(3, "C");
System.out.println("Cache after adding three items: " + cache);
cache.get(1); // Access key 1
cache.put(4, "D"); // This will evict key 2
System.out.println("Cache after accessing key 1 and adding key 4: " + cache);
}
}
6. Find the Missing Number in an Array
Problem: Given an array containing n
distinct numbers taken from 0, 1, 2, ..., n
, find the missing number.
Approach:
This question can be efficiently solved with the Gauss formula for summing integers. Subtract the actual sum of the array from the expected sum to get the missing number.
Sample Solution:
public class MissingNumber {
public static int findMissingNumber(int[] nums) {
int n = nums.length;
int expectedSum = n * (n + 1) / 2;
int actualSum = 0;
for (int num : nums) {
actualSum += num;
}
return expectedSum - actualSum;
}
public static void main(String[] args) {
int[] nums = {3, 0, 1};
System.out.println("Missing number: " + findMissingNumber(nums));
}
}
- java coding test questions and answers
- java coding questions and answers pdf
- java coding interview questions for experienced professionals
- java coding interview questions pdf
- java coding questions for 5 years experience
- java coding questions for beginners
- Popular Java Coding Questions & Answer for 2025
- Popular Java Coding Questions & Answer for 2025
- java coding interview questions for 10 years experience
- java coding questions for practice
- popular java coding questions for freshers
- popular java coding questions and answers
- popular java coding questions for experienced
- Popular Java Coding Questions & Answer for 2025
- Popular Java Coding Questions & Answer
- Popular Java Coding Questions
2 comments