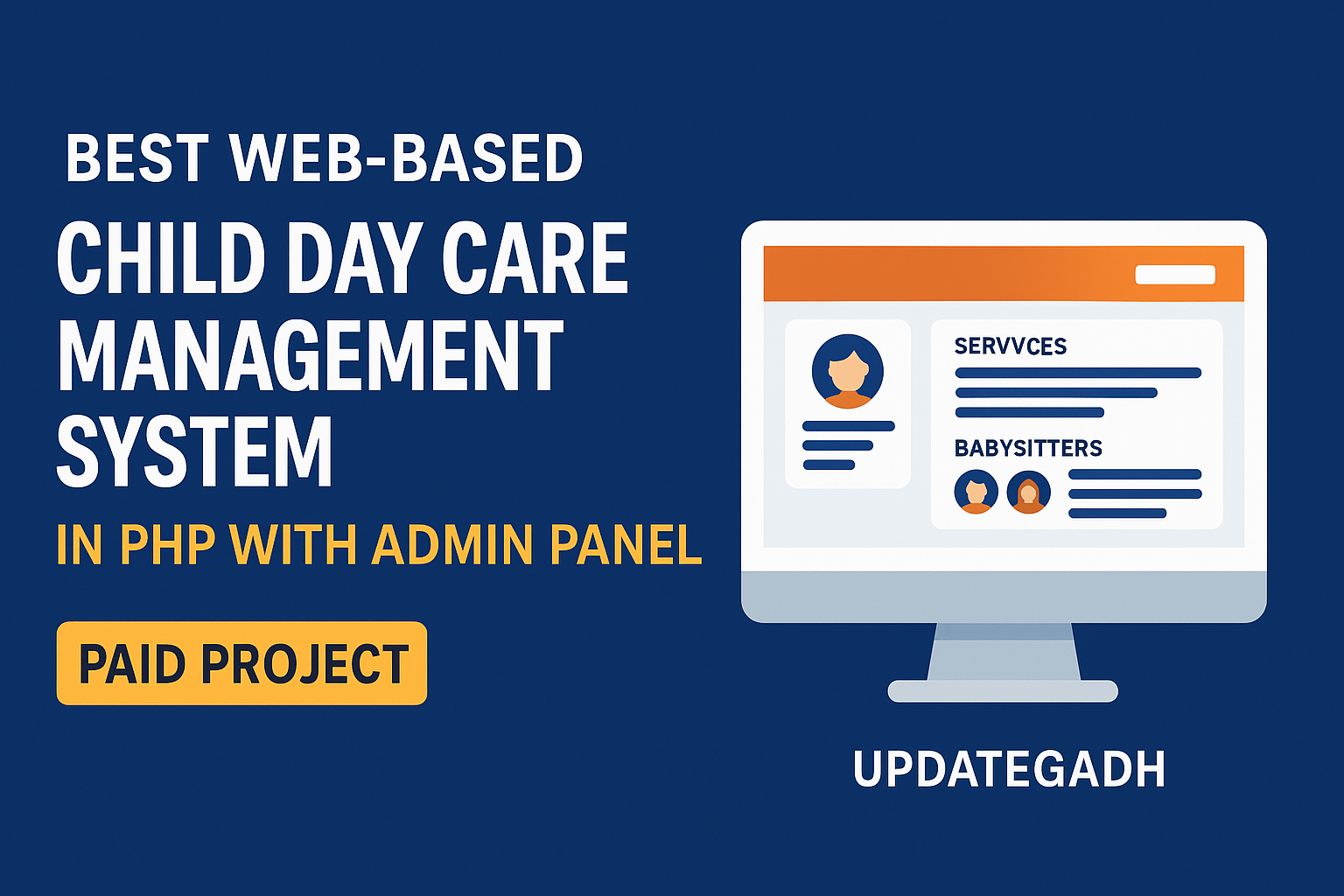
Insurance Management System In Python Free Source Code
Introduction
Insurance, while a necessity in our lives, can often feel overwhelming with its complexities. Managing policies, claims, and customer information efficiently is not only crucial for companies but also for individuals seeking peace of mind. Python, a powerful and intuitive programming language, provides an efficient answer to these problems. By creating an Insurance Management System in Python, we can streamline these processes and make insurance management far more approachable.
INSURANCE MANAGEMENT SYSTEM IN PYTHON
Table of Contents
Features of an Insurance Management System
- Customer Management: Easily track customer details, including personal info, active policies, and claims.
- Policy Management: Add, update, or remove insurance policies with ease.
- Claims Processing: Automate the claims processing flow to minimize errors and speed up the service.
- Report Generation: Provide clients and management with reports on policy statuses, claims, and other key metrics.
- User Authentication and Security: Keep sensitive data secure through proper authentication methods.
Each of these features adds to the overall utility of the system, making it a valuable tool for both insurance companies and policyholders.
Setting Up
Before diving into code, you’ll need to set up a Python development environment. You can use any code editor like Visual Studio Code, PyCharm, or even Jupyter Notebooks. Install Python if you haven’t done so already, and make sure to install necessary libraries such as:
sqlite3
for the databasetkinter
for the UIpandas
for handling data
Open your terminal and use the following commands:
pip install pandas
pip install tk
These libraries will form the backbone of your project, allowing you to manage databases, create a user-friendly interface, and manipulate data.
Database
To manage all the data involved in an insurance system—like customer details, policy information, and claims—you’ll need a reliable database. For simplicity, we’ll use SQLite, which is lightweight and easy to integrate with Python.
import sqlite3
# Connect to database or create it
conn = sqlite3.connect('insurance.db')
# Create a cursor object
c = conn.cursor()
# Create a table for customers
c.execute('''CREATE TABLE IF NOT EXISTS customers (
id INTEGER PRIMARY KEY,
name TEXT,
policy_number TEXT,
claim_status TEXT)''')
conn.commit()
conn.close()
This sets up the basic database structure for customer management, and you can expand it further to include policies and claims.
Basic Structure
Once you have your database in place, it’s time to build the overall system structure. Your system will have multiple modules—like customer management, policy handling, and claims processing.
Here’s a simple structure for your InsuranceManagementSystem.py
file:
class InsuranceManagementSystem:
def __init__(self):
self.customers = []
self.policies = []
def add_customer(self, customer):
self.customers.append(customer)
def add_policy(self, policy):
self.policies.append(policy)
def process_claim(self, customer_id, policy_number):
# Logic for processing a claim
pass
Creating the Policy Module
Inputting New Policies
Every insurance system needs a way to input new policies. You can create a function that lets you input policies manually or through a file upload.
def add_policy(self, policy):
self.policies.append(policy)
Policy Update and Deletion
Your system should also allow for policies to be updated or removed, ensuring the data is always accurate.
def update_policy(self, policy_number, new_details):
# Logic to update policy
pass
Managing Customer Data
One of the most essential parts of an Insurance Management System is keeping customer data secure and up to date. The system should allow you to:
- Add new customers
- View customer details
- Update customer information
- Delete customer records
Here’s a simple function to add a customer:
def add_customer(self, customer):
self.customers.append(customer)
Claims Processing Module
Processing claims can be one of the most time-consuming tasks in insurance management. Automating this step ensures a smoother experience for both the client and the business.
def process_claim(self, customer_id, policy_number):
# Verify claim and update status
pass
Generating Reports
To help management or clients see the bigger picture, your system should be able to generate reports about active policies, pending claims, or customer histories.
def generate_report(self):
# Generate a summary of policies and claims
pass
Integrating Error Handling and Security
Security is paramount in any system dealing with sensitive data. Python offers various ways to secure your data through encryption and proper user authentication.
import hashlib
def hash_password(password):
return hashlib.sha256(password.encode()).hexdigest()
Adding a User Interface (UI)
While your system can work just fine through the command line, adding a user-friendly interface is a huge bonus. Using tkinter
, you can create a simple interface for inputting and retrieving information.
import tkinter as tk
root = tk.Tk()
root.title("Insurance Management System")
# Add UI elements here
root.mainloop()
Source Code for Insurance Management System
Below is a simplified version of the source code:
import sqlite3
import tkinter as tk
# Your Insurance Management System code goes here
Testing and Debugging
No system is complete without thorough testing. Make sure to test each function and fix any bugs you encounter. This not only ensures the system works well but also gives you confidence in your creation.
Future Enhancements and Final Thoughts
The beauty of coding is that you can always improve. Future enhancements could include cloud integration, adding advanced security features, or even AI-driven claims processing. The possibilities are endless.
In building this Insurance Management System, you’re not just writing code—you’re creating something that has the potential to make lives easier. That’s the emotional heart of coding. You’re solving real-world problems, one line at a time.
- New Project :-https://www.youtube.com/@Decodeit2
- PHP PROJECT:- CLICK HERE
- insurance management system in python
- insurance management system project in python
- INSURANCE MANAGEMENT SYSTEM IN PYTHON
- insurance management system
- insurance python project
- insurance management system project source code
- python in insurance
- python project management library
- insurance python project with source code
- INSURANCE MANAGEMENT SYSTEM IN PYTHON FREE SOURCE CODE
2 comments